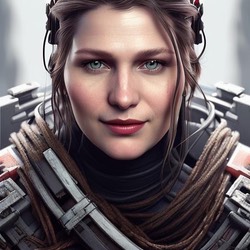
Demystifying User-Defined Type Guards in TypeScript
You know you're in the big leagues of TypeScript when you start talking about user-defined type guards. It's like the VIP section of type safety, where you get to be the bouncer deciding who gets in and who doesn't. In this wild world of TypeScript, user-defined type guards are your secret weapon. They're like the precision-guided missiles of typing, ensuring that your variables are exactly what you expect them to be. So, grab your virtual bouncer suit, because we're about to dive into the fancy world of user-defined type guards!
Purpose of User-Defined Type Guards
In the world of TypeScript, user-defined type guards are like the vigilant gatekeepers of a grand event. Their main goal is to ensure that only the right types gain entry. They're the discerning judges, meticulously examining variables to confirm their true identity. By implementing these custom guards, you're essentially setting up a strict guest list for your code. This tailored approach to typing is your key to elevating the overall security and reliability of your TypeScript projects.
This article delves into concrete examples where user-defined type guards shine. Through cases like validating inputs and managing dynamic data, we see their crucial role in maintaining type integrity.
Type Predicate Functions: The Art of Defining Types
Crafting robust type definitions is an art, and Type Predicate Functions are the master strokes that lend impeccable definition to your code. These functions act as keen evaluators, accurately inspecting variables for their true nature. This section shows how leveraging Type Predicate Functions elevates the overall quality and dependability of your TypeScript projects.
Example 1: Filtering VIP Cats
Consider a scenario where you have an array of different types of cats, each with its own set of attributes. You want to filter out only the "fancy" cats based on specific criteria.
interface Cat {
name: string;
breed: string;
accessories?: string[];
}
interface FancyCat extends Cat {
accessories: string[];
}
function isFancyCat(cat: Cat): cat is FancyCat {
return !!cat.accessories && cat.accessories.length > 0;
}
const cats: Cat[] = [
{ name: 'Whiskers', breed: 'Siamese', accessories: ['Bow Tie', 'Top Hat'] },
{ name: 'Fluffy', breed: 'Persian' },
{ name: 'Mittens', breed: 'Tabby', accessories: ['Diamond Collar'] }
];
const fancyCats = cats.filter(isFancyCat);
Here, the isFancyCat
function serves as the bouncer, allowing only the "fancy" cats with accessories to enter the VIP lounge. On a more serious note, we've explored the use of user-defined type guards to distinguish between regular cats and their more sophisticated counterparts, the FancyCats. By employing the isFancyCat
function, we've effectively narrowed down the types based on the presence of accessories, enhancing our code's precision.
This technique proves invaluable when dealing with complex data structures, where different objects may have distinct properties. With user-defined type guards, we can confidently and accurately categorise these objects, ensuring that our code operates with maximum clarity and reliability.
Example 2: Handling Specialised Data Structures
Suppose you're working with a specialised data structure that can have varying shapes based on certain conditions. You want to ensure that the structure adheres to the expected shape before processing it.
type DataStructureA = { kind: 'A'; value: number };
type DataStructureB = { kind: 'B'; message: string };
type DataStructure = DataStructureA | DataStructureB;
function isDataStructureA(data: DataStructure): data is DataStructureA {
return data.kind === 'A';
}
function processData(data: DataStructure) {
if (isDataStructureA(data)) {
// Process data as type A
console.log(`Processing data of kind A with value: ${data.value}`);
} else {
// Process data as type B
console.log(`Processing data of kind B with message: ${data.message}`);
}
}
const dataA: DataStructure = { kind: 'A', value: 42 };
const dataB: DataStructure = { kind: 'B', message: 'Hello World' };
processData(dataA);
processData(dataB);
In this case, we have a DataStructure
type that can take two different shapes: one with a kind
of 'A' and a value
of number, and another with a kind
of 'B' and a message
of string.
The isDataStructureA
function acts as our type guard, allowing us to discern whether a given DataStructure
object is of type 'A' or 'B'. This ensures that we can confidently apply specialised logic to each type without encountering type errors.
Example 3: Validating User Inputs
Imagine you're building a form validation system where different input types have varying validation criteria. You want to ensure that each input type is validated correctly before submission.
interface TextInput {
type: 'text';
value: string;
}
interface NumberInput {
type: 'number';
value: number;
}
type Input = TextInput | NumberInput;
function isValidTextInput(input: Input): input is TextInput {
return input.type === 'text' && input.value.length > 0;
}
function isValidNumberInput(input: Input): input is NumberInput {
return input.type === 'number' && !isNaN(input.value);
}
function validateInput(input: Input) {
if (isValidTextInput(input)) {
console.log(`Valid text input: ${input.value}`);
} else if (isValidNumberInput(input)) {
console.log(`Valid number input: ${input.value}`);
} else {
console.log('Invalid input');
}
}
const textInput: Input = { type: 'text', value: 'Hello' };
const numberInput: Input = { type: 'number', value: 42 };
validateInput(textInput);
validateInput(numberInput);
In this scenario, we have two types of inputs: TextInput
and NumberInput
. Each type has its own specific validation criteria.
The functions isValidTextInput
and isValidNumberInput
act as type guards, allowing us to determine whether a given input is of type TextInput
or NumberInput
. This enables us to apply the appropriate validation logic based on the type of input provided.
By utilising user-defined type guards, we ensure that each input type is validated correctly before further processing. This approach enhances the reliability and accuracy of our form validation system, particularly in situations where different input types have distinct validation requirements.
Assertion Functions: Asserting Types with Authority
TypeScript's Assertion Functions are a force to be reckoned with when it comes to type validation. In contrast to Type Predicate Functions, which verify types based on conditions, Assertion Functions assert that a variable unequivocally belongs to a particular type. They offer a level of confidence that goes beyond mere checks. This segment delves into the strengths of Assertion Functions and provides insights into how they differ from Type Predicate Functions, adding an extra layer of certainty to your typing toolkit.
Example 1: Asserting Fancy Cats
Let's slightly modify the Fancy Cats example from the Type Predicate Functions section to work with Assertion Functions instead.
interface Cat {
name: string;
breed: string;
accessories?: string[];
}
interface FancyCat extends Cat {
accessories: string[];
}
function assertFancyCat(cat: Cat): asserts cat is FancyCat {
if (!('accessories' in cat)) {
throw new Error(`This cat is not fancy enough to enter the VIP lounge.`);
}
}
const myCat: Cat = { name: 'Whiskers', breed: 'Siamese', accessories: ['Bow Tie', 'Top Hat'] };
assertFancyCat(myCat);
// Now myCat is treated as FancyCat
const fancyAccessories = myCat.accessories;
Unlike Type Predicate Functions, which narrow down types based on conditions, the assertFancyCat
function takes a more assertive stance. It boldly declares that a given cat is of type FancyCat
by ensuring the presence of accessories. This approach provides an extra layer of certainty in typing, going beyond mere checks to enforce precise type expectations. If a cat doesn't meet the criteria, an error is thrown, reflecting the strict assertion nature of this function.
Example 2: Asserting Specialised Data Structures
This is the same example from the Type Predicate Functions section modified in a way to better showcase how assertion functions work.
type DataStructureA = { kind: 'A'; value: number };
type DataStructureB = { kind: 'B'; message: string };
type DataStructure = DataStructureA | DataStructureB;
function assertDataStructureA(data: DataStructure): asserts data is DataStructureA {
if (data.kind !== 'A') {
throw new Error(`This data structure is not of kind 'A'.`);
}
}
const myData: DataStructure = { kind: 'A', value: 42 };
assertDataStructureA(myData);
// Now myData is treated as { kind: 'A', value: number }
const processedValue = myData.value;
Here, Assertion Functions take center stage in ensuring the correct categorisation of data structures. The assertDataStructureA
function goes beyond traditional checks by explicitly asserting that the provided data structure is of kind 'A'. This approach differs from Type Predicate Functions, which focus on narrowing down types based on conditions. With Assertion Functions, we're not merely verifying; we're asserting a specific type, providing a higher level of confidence in our type declarations.
Example 3: Asserting Valid Inputs
Similarly as above, this is also an equivalent of the Type Predicate Functions example modified to work with assertion functions.
interface TextInput {
type: 'text';
value: string;
}
interface NumberInput {
type: 'number';
value: number;
}
type UserInput = TextInput | NumberInput;
function assertValidTextInput(input: UserInput): asserts input is TextInput {
if (input.type !== 'text' || typeof input.value !== 'string') {
throw new Error(`Invalid text input.`);
}
}
const userInput: UserInput = { type: 'text', value: 'Hello' };
assertValidTextInput(userInput);
// Now userInput is treated as TextInput
const stringValue = userInput.value;
In this example, we showcase how Assertion Functions excel in validating user inputs. The assertValidTextInput
function asserts that a given input is of type 'text', offering a level of certainty that surpasses the traditional checks performed by Type Predicate Functions. This approach ensures that we're working with valid text inputs, leaving no room for ambiguity. If the input doesn't meet the specified criteria, an error is thrown, underscoring the assertive nature of this type guard.
Combining Both Techniques
interface Animal {
type: string;
name: string;
wingspan?: number;
swimDepth?: number;
}
interface Bird extends Animal {
type: 'bird';
wingspan: number;
}
interface Fish extends Animal {
type: 'fish';
swimDepth: number;
}
function isBird(animal: Animal): animal is Bird {
return animal.type === 'bird';
}
function isFish(animal: Animal): animal is Fish {
return animal.type === 'fish';
}
function assertBirdWithLargeWingspan(animal: Animal): asserts animal is Bird {
if (isBird(animal) && animal.wingspan > 2) {
return;
}
throw new Error(`This is not a bird with a large wingspan.`);
}
const zoo: Animal[] = [
{ type: 'animal', name: 'Lion' },
{ type: 'bird', name: 'Eagle', wingspan: 2.5 },
{ type: 'bird', name: 'Sparrow', wingspan: 0.2 },
{ type: 'fish', name: 'Trout', swimDepth: 10 },
{ type: 'fish', name: 'Goldfish', swimDepth: 1 },
];
const birds: Bird[] = zoo.filter(isBird);
birds.forEach(assertBirdWithLargeWingspan);
const largeWingspanBirdNames: string[] = birds.map((bird) => bird.name);
In this example, we have a zoo with various animals, some of which are birds and fish. We use both Type Predicates (isBird
and isFish
) and an Assertion Function (assertBirdWithLargeWingspan
) to perform advanced type narrowing.
First, we filter the animals to get an array of only birds using isBird
. Then, we iterate through the birds and use assertBirdWithLargeWingspan
to assert that each bird has a wingspan greater than 2 units. If the assertion fails, an error is thrown.
Finally, we extract the names of birds with large wingspans. This example showcases how Type Predicates and Assertion Functions can be combined to perform complex type narrowing and validation in TypeScript, ensuring precise typing and robust error handling.
Pitfalls to Watch Out For
Creating user-defined type guards can be a powerful tool, but it's not without its potential pitfalls. One such pitfall is overly complex type guard logic, which can make the code hard to understand and maintain. Another common mistake is forgetting to include the is
keyword in the return type of the type guard function. Yet another mistake is forgetting to return a boolean value in the type guard function. These simple omissions can lead to unexpected type narrowing. To sidestep these issues, keep your type guards clear and concise, and always double-check your return types.
Conclusion...use type guards because safety is the coolest accessory!
So there you have it! User-defined type guards are like the vigilant gatekeepers of the TypeScript world, ensuring that only the right guests enter the VIP lounge. They're not just fancy jargon; they're your ticket to a safer, more robust codebase.
Remember, these guards are your trusty sidekicks in the quest for type safety. But like any good sidekick, they have their quirks. Watch out for common pitfalls, like forgetting that all-important boolean return, and steer clear of overly complex logic.
With user-defined type guards in your toolkit, you'll be navigating the TypeScript realm with confidence, knowing that your types are in good hands. So go ahead, let those guards do their thing, and code on with style and safety!